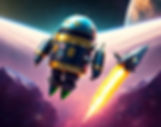
Data binding is an indispensable element in creating today's UI. It permits coders to latch design components onto the data inside their program's dataset, making it possible that any switch to the data reflects directly on the user interface. Google's declarative UI collection of tools, Jetpack Compose, carries several procedures to make use of data binding in your software solution. In this write-up we will check out various approaches of putting into practice data binding with Jetpack Compose and uncover it.
1. ViewModel and LiveData
The ViewModel and LiveData are pillars found in the Android Architecture Components, consisting of a set of tools and rules meant to produce more durable, feasible, and uncomplicated apps. The ViewModel maintains the app’s collection of information while the LiveData makes it possible for the UI to be aware of any new alterations to that data.
To use ViewModel and LiveData in Jetpack Compose, you need to create a ViewModel class that holds your app's data and expose LiveData objects that emit changes to that data. Then, you can use the observeAsState function to observe changes to the LiveData objects and trigger recomposition of the UI.
Here's an example of how to use ViewModel and LiveData in Jetpack Compose:
class MyViewModel : ViewModel() {
private val data = MutableLiveData<String>()
fun setData(newData: String) {
data.value = newData
}
}
@Composable
funMyScreen(viewModel: MyViewModel = viewModel()) {
val data by viewModel.data.observeAsState("")
Text(text = data)
}
In this example, a class called "MyViewModel" contains a variable of type 'LiveData' denoted as 'data', along with a 'setData' function that modifies the value. The component inside our app, known as ‘MyScreen’ implements something known as the ‘observeAsState’ feature to monitor alterations within this particular LiveData object. Later on, any transformed data will be delivered towards the element utilized to demonstrate the info.
2. State
The Compose state function allows developers to incorporate decisions that can be manipulated in Compose functions. Defining a piece of data applicable to your User Interface explains the relevance of the added state; which has the power to determine details associated with UI gadgets and control how they appear. As App production develops, any amendments made in regards to your configuration results in an entirely schematized recreation sourced from Compose – demonstrated within this snippet concerning usages of state in Jetpack Composing.
@Composable
funMyScreen() {
val (data, setData) = remember { mutableStateOf("") }
TextField(
value = data,
onValueChange = { newData ->
setData(newData)
}
)
Text(text = data)
}
In this example, we use the remember function to construct a mutable state variable data that holds a string value. We also define a setData function that changes the data variable with a new value.
We utilise the TextField composable in the MyScreen composable method to let the user enter a new value for the data. you, but you, and more, in the it.. of the other. of the an of the the of the of the of the of,. of the.. ofamore. Lastly, we use the Text composable to display the data's current value.
Creating and managing state for a UI is advantageous in its simplicity; however, doing so can lead to greater cohesion between the interface and its data, potentially leading to difficulty with testing and sustainability.
3. Flow
Kotlin coroutines provide a powerful and flexible way to handle asynchronous operations in your app. Jetpack Compose builds on top of coroutines to provide a reactive programming model using the Flow class.
A Flow is an asynchronous stream of data that emits values over time. You can use the collectAsState function to observe changes to the flow and trigger recomposition of the UI.
Here's an example of how to use Flow in Jetpack Compose:
fun getData(): Flow<String> = flow {
emit("Initial value")
delay(1000)
emit("New value")
}
@Composable
fun MyScreen() {
val data by getData().collectAsState(initial = "")
Text(text = data)
}
In the above example we have written a getData function which returns a flow of string that emits an initial value and then a delay of seconds and then emit a new value.
In MyScreen which is a UI collect stage we collect the data as a state and show it to our screen.
Take Away Points:
Finally, data binding is a crucial component of contemporary UI development, and Jetpack Compose offers a variety of ways to include data binding into your app. For a clear separation of concerns, you can use ViewModel and LiveData, state for a straightforward and user-friendly method, or Flow for a reactive programming model.
The optimal strategy will rely on your app's design and requirements because each technique has advantages and disadvantages of its own. With Jetpack Compose's data binding feature, you can create reactive, dynamic user interfaces that instantly adapt in reaction to changes in the data model of your project.